WELCOME TO ELECTRICKS MAGIC
State of the Art Mentalism Magic
We make high-quality, reliable magic tools with one-of-a-kind integrations.
Our devices are not only compatible with each other, but also with other popular products on the market. We work closely with fellow product creators, app developers and magicians to provide you a unique experience unavailable elsewhere.
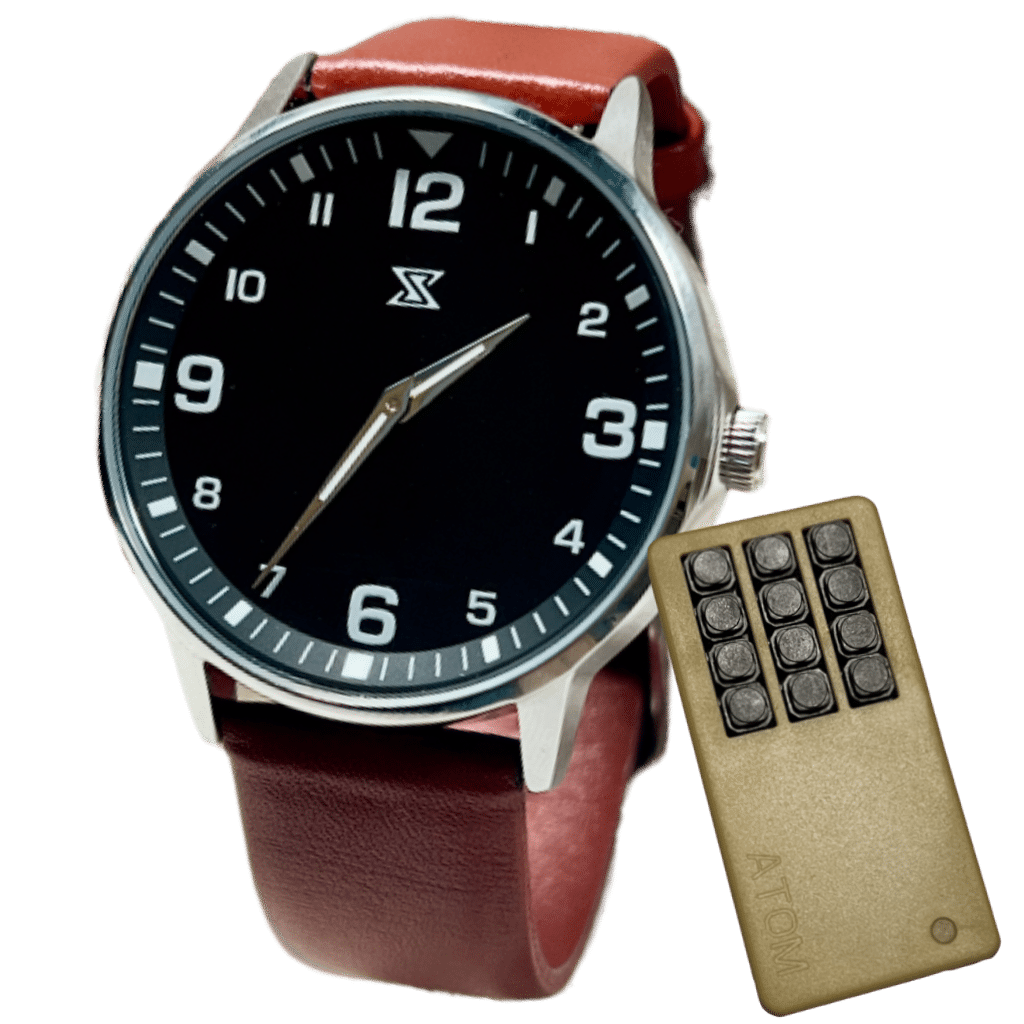
Best Sellers
WE ARE PROUD OF OUR
SB Watch 2, Atom 2, PeekSmith 3 Bundle
NEW KIDS ON THE BLOCK
New Arrivals
SB Watch 2 (2024)
$497
- A prediction watch AND a peek device in one
- Innocent look, has a sleek design and natural aesthetics
- Comes with the TimeSmith app and many routines
The Ultimate Prediction and Peek Watch
Control the time with our elegant analog wristwatch with peek functionality.
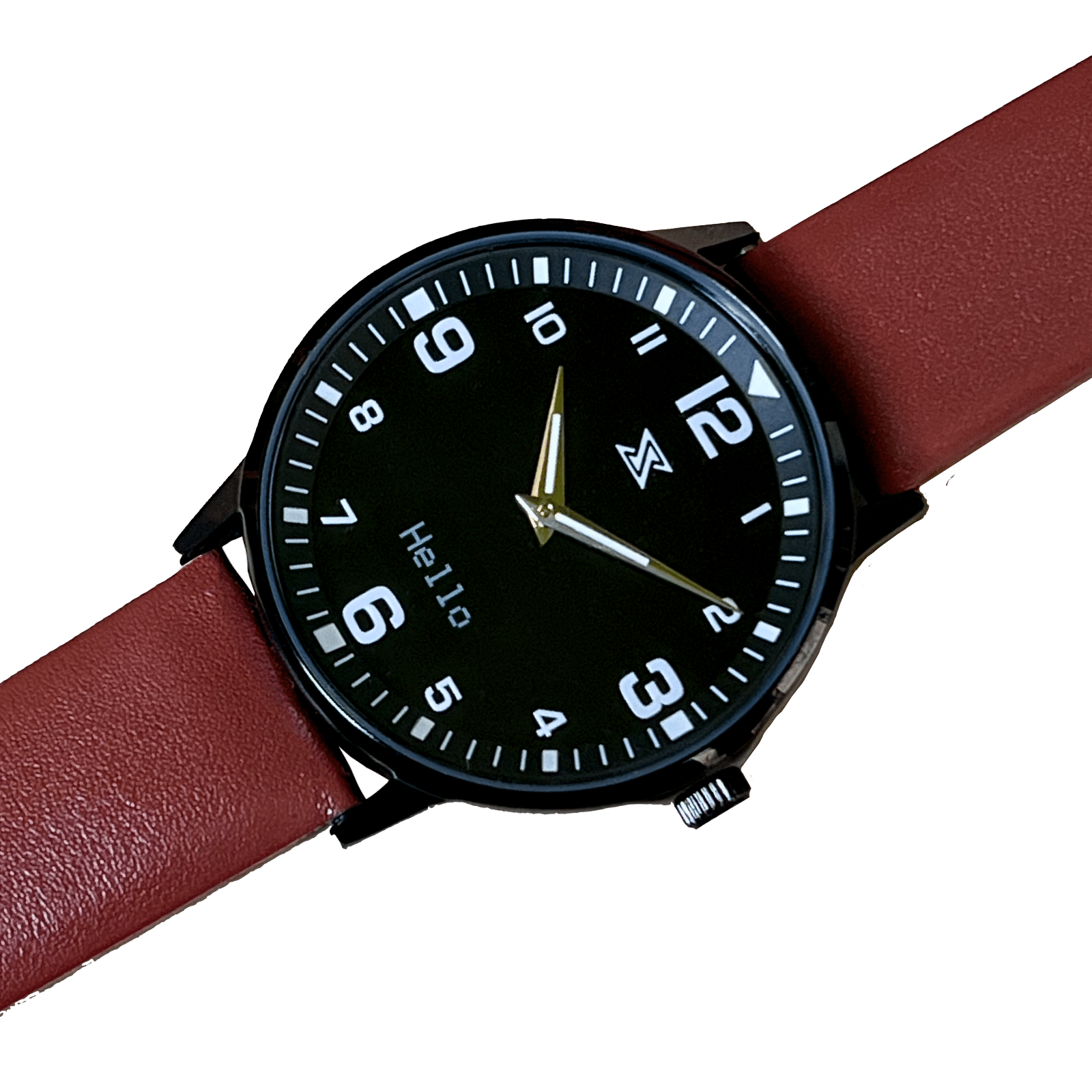
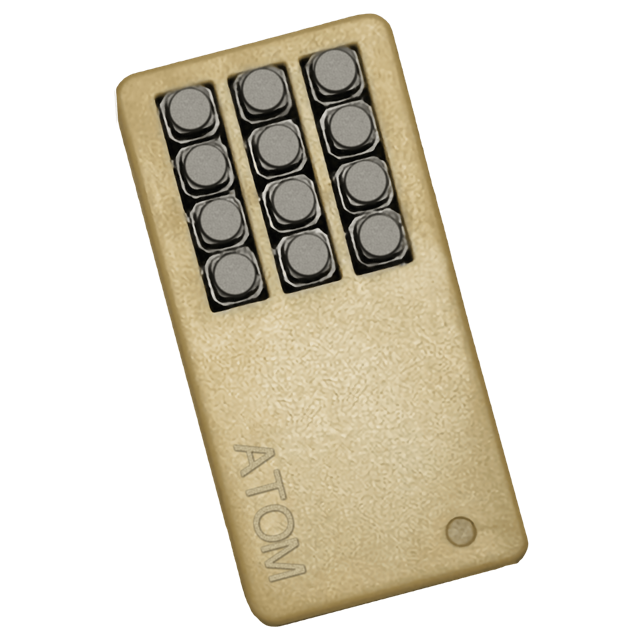
Atom 2 Smart Remote
$247
- Compact and Powerful Design
- Direct SB Watch and PeekSmith 3 Communication
- MagiScript Support with Endless Possibilities
The 12 Button Magic Wand
Atom 2 is a game-changing product of Electricks. Our intelligent remote is designed to redefine how you interact with your electronic devices. The 12 silent buttons, vibration motor, RGB LED, and revolutionary MagiScript integration allow you to run custom mini-apps, making Atom 2 not just a remote but a platform.
On Sale
GRAB THEM NOW
Traditional Pocket Watches (2 Models)
SB Watch 2, Atom 2, PeekSmith 3 Bundle
Prediction Watches
TIME MANAGEMENT
Traditional Pocket Watches (2 Models)
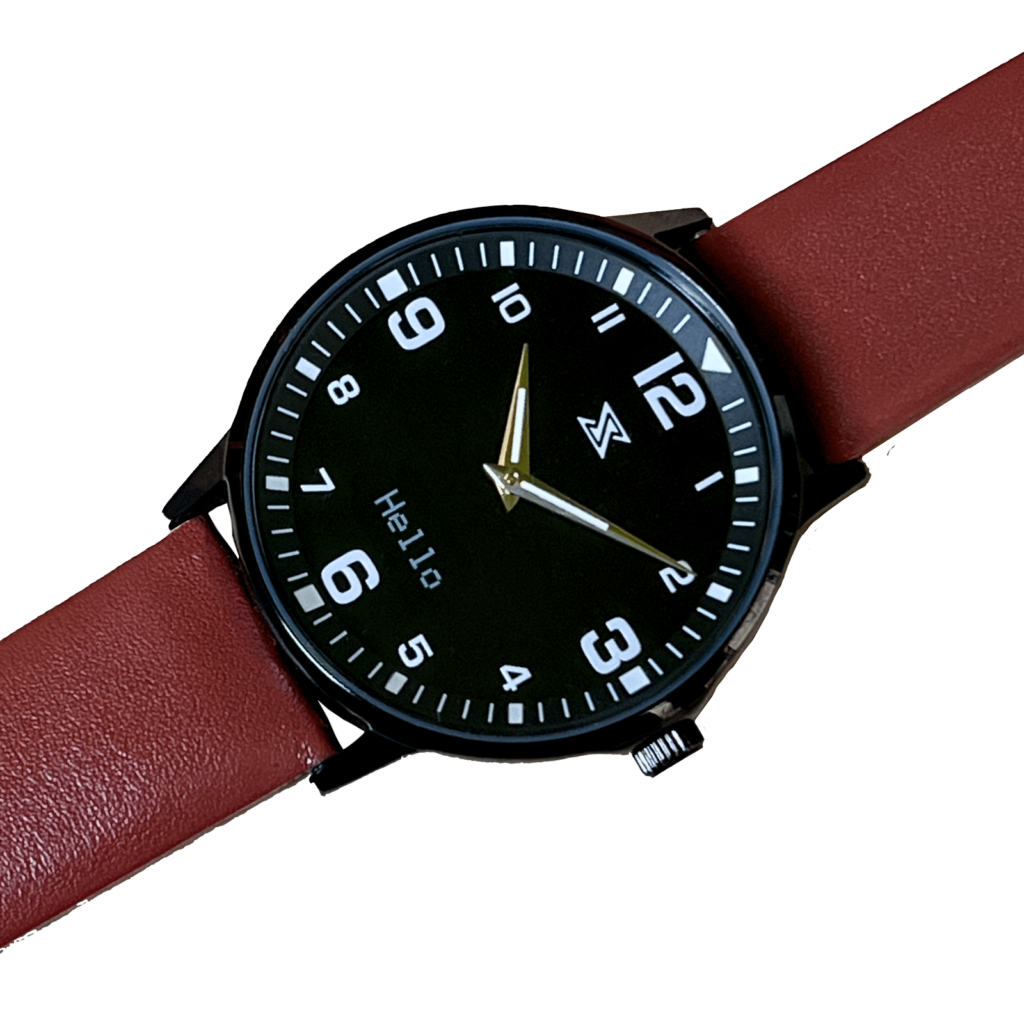
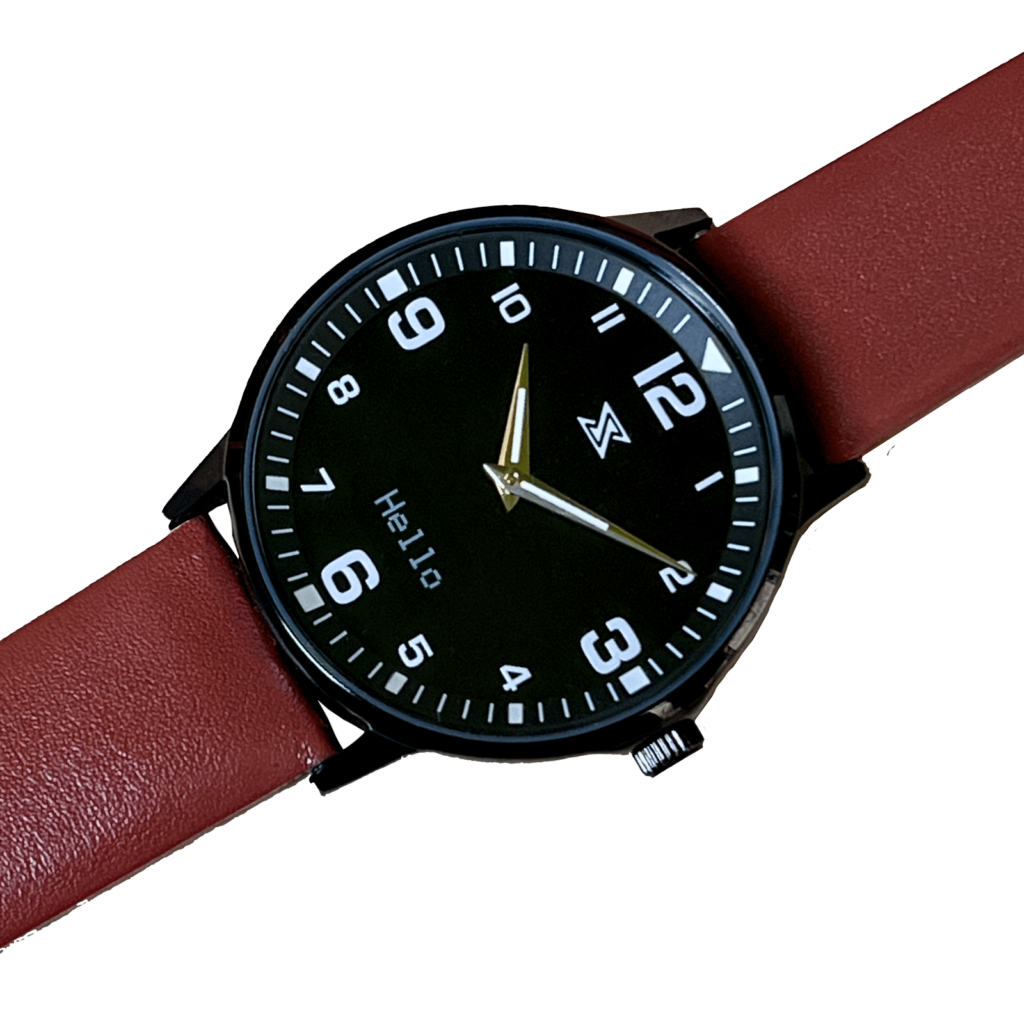
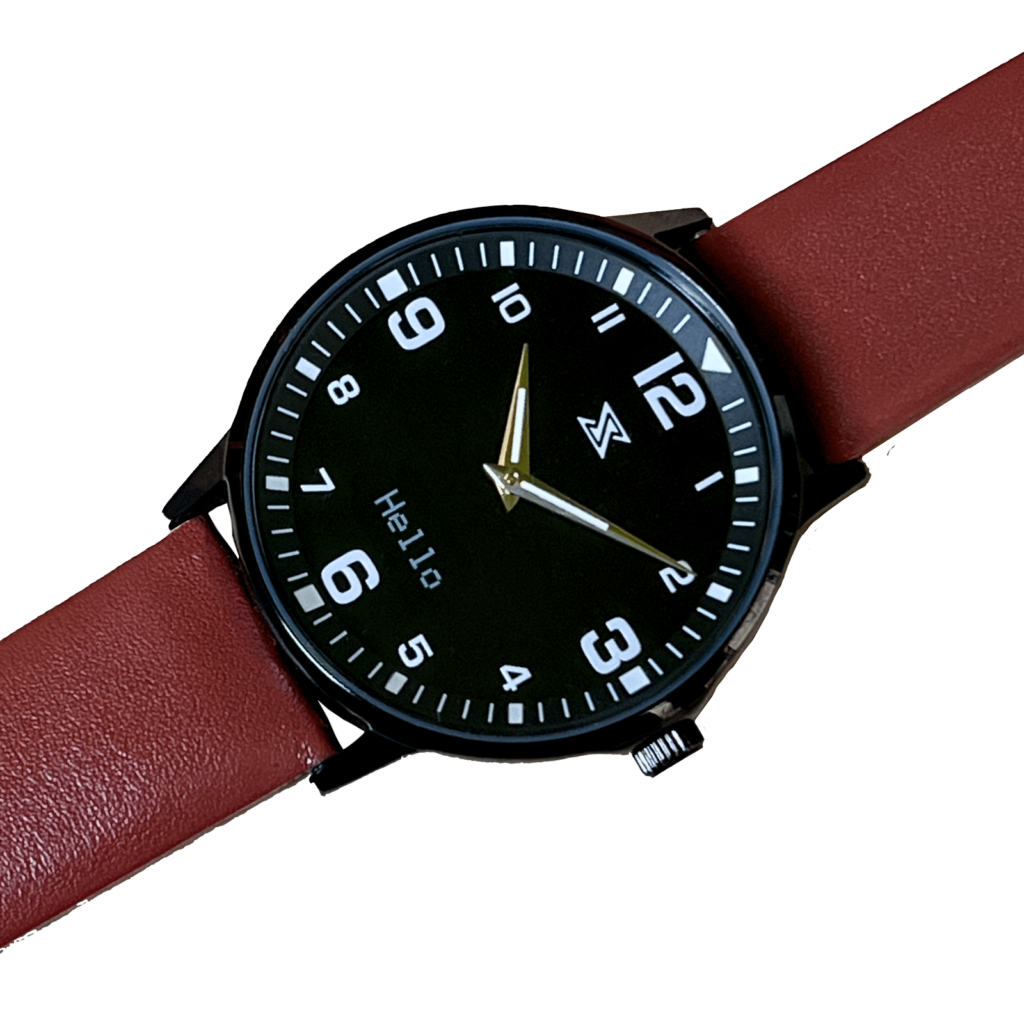
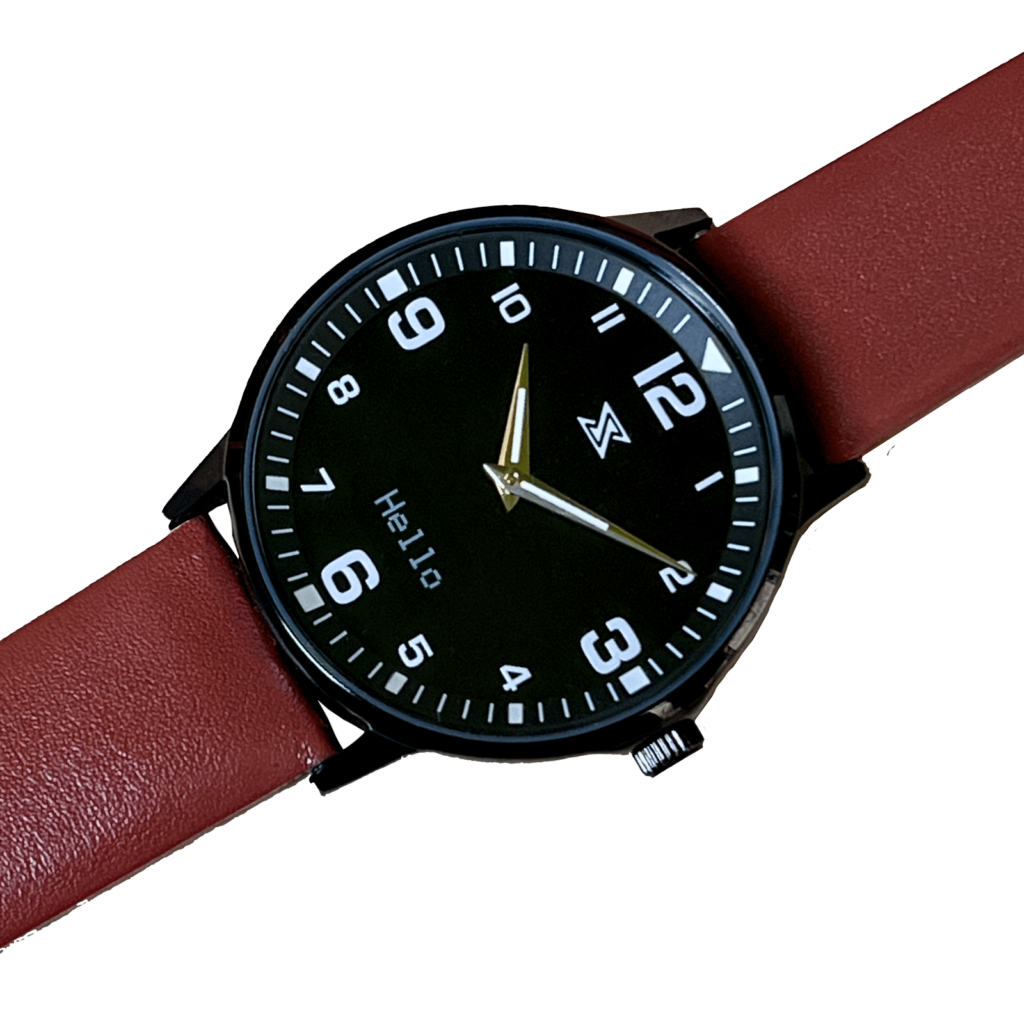
Quality Tools for Every Magician
Magicians love our products





















NEWSLETTER
Innovation on the horizon
Electricks is a rising star in the world of magic, renowned for designing cutting-edge electronic products and unparalleled accessories for magicians of all levels.
We are two creators:
András Bártházi (Electricks – hardware & firmware)
Benke Smith (freelancer – apps)
Electricks Kft.
Address: Bánki Donát utca 73. IV/1.
City: Budapest
Post Code: 1148
Country: Hungary
VAT Number: 29305885-2-42
- Useful Information
- Facebook Communities
Join us for support, tips, and ideas.
Electricks & Magic Apps by Benke
PeekSmith, CubeSmith, SBWatch and TimeSmith, Spotted Dice and DiceSmith, Atom, MagiScript, ScaleSmith, Impression Pad Support
❤️ Made with Love
© Copyright 2023 Electricks Kft.
All rights reserved. Powered by Speedmarketing