Atom Level
About
Did you know that PeekSmith 3 has an accelerometer inside? Let us demonstrate with this code how you can use it to create a Level Tool. For sure it is for fun, however, you will get some idea of how you can work with accelerometer data. Atom andΒ SB Watch also has an accelerometer!
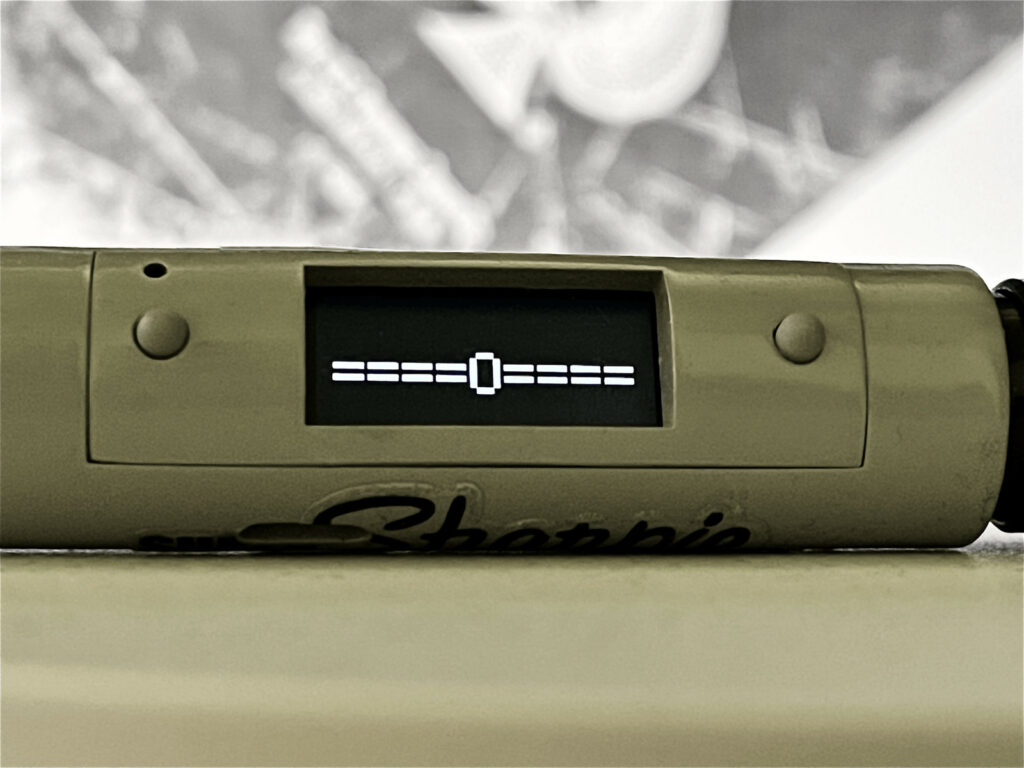
We are not going to complicate the project. At first, in the main function, the code connects to a PeekSmith, then turns on the accelerometer, and starts a timer to run the vibrate function once per second.
In the onEvent function, it returns if the event is not about accelerometer data from the PeekSmith accelerometer. Then convert the string to 3 numbers, and call the onAccel method with them.
In the onAccel function, we only need the x value. It is transformed into a number between 0 and 8, and the code prints the “graphics” to the PeekSmith screen.
And finally, the vibrate function, which is running every second, the code checks if the level is middle. If yes, it sends a short vibration to PeekSmith.
function main() {
ps.connect();
ps.print('Atom\nLevel');
ps.accel('on');
setInterval(vibrate, 1000);
}
function onEvent(e) {
if (e.type !== 'xyz' || e.source !== 'ps:accel') return;
let xyz = strSplit(e.value, ',');
for (let index = 0; index < 3; index++) {
xyz[index] = parseInt(xyz[index]);
}
onAccel(xyz[0], xyz[1], xyz[2]);
}
let currentLevel = -1; // unknown
let levels = [
'O--------',
'-O-------',
'--O------',
'---O-----',
'====O====',
'-----O---',
'------O--',
'-------O-',
'--------O',
];
function onAccel(x, y, z) {
currentLevel = ( (x / 2048) + 1) * 4.2 | 0; // calculate a number between 0 - 8
if (currentLevel < 0) currentLevel = 0;
if (currentLevel > 8) currentLevel = 8;
ps.print(levels[currentLevel]);
}
function vibrate() {
if (currentLevel === 4) ps.vibrate('.');
}
General
π First Steps
MagiScript Editor
π Basics
π Keyboard Shortcuts
π Running Your First Program
π App Store
π Debugging Techniques
Examples
π Atom Time
ποΈ Time Practice
π Atom Drum
ποΈ Atom Stack
π Atom Square
π Atom Level
π Atom THMPR
π Poker Hands
π Keyboard Numeric
π Keyboard NOKIA
π Keyboard Cursor
π Keyboard Media
π Keyboard Custom
Input/Output
π Buttons
π Vibration Motor
π RGB LED
π Devices
π PeekSmith
π Quantum
π Teleport
π Spotted Dice
π SB Watch
π IARVEL Watch
π Fossil Watch
π Cosmos Printer
π PeriPage Printer
π ATC Remote
π Labko Scrabble
π Bluetooth Keyboard
π Bluetooth Mouse
π Timers
π Database
π Events
π System (exit, sleep, rand)
π Objects (card, time)
Language
π Summary
π Comments
π Operators
π Control Flow
π Functions
π Numbers
π Strings
π Arrays
π Objects
π Uint8Array